As a budding programmer, understanding modern application architecture is crucial. The three-tier application architecture is a robust design pattern that not only organizes your application’s components but also provides multiple layers of security and scalability. Whether you’re building a desktop app, mobile application, or web service, this architecture offers a solid foundation for creating secure and efficient software.
The ongoing discussions whether to apply microservices or monolith architecture doesn’t change much in this regard. It usually only affects the server side of your solution. There are still clients, some server and some data to store.
What is Three-Tier Architecture?
Three-tier architecture divides an application into three distinct layers:
- Presentation Layer (Client Tier)
- Application Layer (Business Logic Tier)
- Data Layer (Database Tier)
Let’s dive deep into each layer and explore how they work together to create a secure and efficient application.
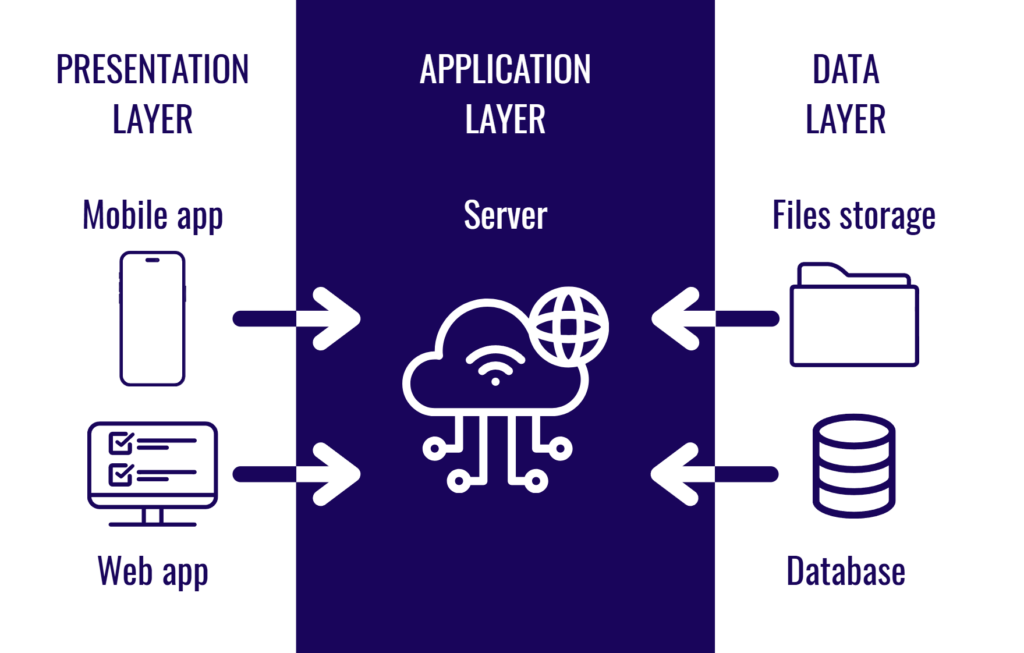
1. Presentation Layer (Client Tier)
This is the user-facing component of your application. It can take multiple forms:
- Web browsers
- Mobile applications (iOS, Android)
- Desktop applications (Windows, macOS, Linux)
Security Considerations:
- Never trust user input
- Implement client-side validation as a first line of defense
- Use HTTPS for web applications
- Implement proper authentication mechanisms
- Minimize sensitive data storage on the client-side
Code Example (Client-Side Validation):
function validateLoginForm(username, password) {
// Client-side validation
if (username.length < 3) {
showError("Username too short");
return false;
}
if (password.length < 8) {
showError("Password must be at least 8 characters");
return false;
}
// Send to server for final authentication
return sendLoginRequest(username, password);
}
2. Application Layer (Business Logic Tier)
This layer sits between the client and the database, processing data, applying business rules, and managing application logic. It acts as a critical security buffer.
Key Responsibilities:
- Authentication and authorization
- Data validation
- Business rule enforcement
- Communication between client and database
Security Mechanisms:
- Implement role-based access control (RBAC)
- Use server-side validation
- Encrypt sensitive data
- Implement proper error handling without exposing system details
Code Example (Authorization Middleware):
def authorize_user(user, required_role):
# Check if user has necessary permissions
if user.role not in required_role:
raise UnauthorizedAccessException("Insufficient permissions")
# Proceed with request if authorized
return process_request()
3. Data Layer (Database Tier)
The final tier stores and manages application data. It’s the most sensitive part of your application and requires robust security measures.
Security Best Practices:
- Use parameterized queries to prevent SQL injection
- Implement least privilege database accounts
- Encrypt data at rest
- Use database-level access controls
- Regularly audit and rotate credentials
Code Example (Secure Database Connection):
def connect_to_database():
# Use environment variables for credentials
connection = psycopg2.connect(
host=os.getenv('DB_HOST'),
database=os.getenv('DB_NAME'),
user=os.getenv('DB_USER'),
password=os.getenv('DB_PASSWORD'),
# Use SSL/TLS for connection
sslmode='require'
)
return connection
Data Flow and Security Considerations
- Client Initiates Request
- Performs initial client-side validation
- Sends request via secure channel (HTTPS)
- Application Layer Processes Request
- Validates user authentication
- Checks authorization levels
- Performs server-side validation
- Sanitizes input data
- Database Interaction
- Executes query with minimal privileges
- Returns only authorized data
- Logs access attempts
Additional Security Recommendations
- Implement multi-factor authentication
- Use JSON Web Tokens (JWT) for stateless authentication
- Apply rate limiting to prevent brute-force attacks
- Keep all software components updated
- Use secure, up-to-date encryption standards
Conclusion
Three-tier architecture provides a scalable, secure framework for building applications across different platforms. By understanding and implementing proper security measures at each layer, you can create robust software that protects both user data and system integrity.
Remember, security is not a one-time implementation but an ongoing process of monitoring, updating, and improving your application’s defenses.
Happy and Secure Coding!